Laravel has become a go-to framework for web development in the PHP world, thanks to its robust features and elegant syntax. But did you know that Laravel can also be a powerful tool for building modern applications using the concept of microservices?
In today’s software landscape, microservices architecture is gaining significant traction. It allows developers to break down complex applications into smaller, independent services. Each service has a well-defined purpose and communicates with others through APIs. This approach offers numerous advantages, including increased agility, improved maintainability, and enhanced scalability.
This blog post delves into the exciting world of Laravel microservices. We’ll explore how Laravel, with its modular design and built-in functionalities, can be leveraged to create a robust microservices architecture. We’ll unpack the core concepts of this architecture, highlight its benefits, and guide you through building microservices with Laravel Lumen, a lightweight Laravel sibling specifically designed for this purpose.
Laravel as Microservice
Laravel itself, with its well-organized structure and rich set of features, can be considered a strong foundation for building microservices. Here’s why:
Modular Design
Laravel is built upon a modular concept, where functionalities like routing, authentication, and database access are offered as independent components. This aligns perfectly with the microservices architecture principle of breaking down applications into smaller, focused services.
Built-in Functionalities
Laravel provides a wealth of built-in functionalities that can be readily utilized within microservices. These include:
- Routing: Laravel’s robust routing system allows for efficient handling of API requests within each microservice.
- Queues: Queues enable asynchronous communication between microservices, ensuring smooth processing of tasks without blocking requests.
- Events: Laravel’s event system facilitates communication and data exchange between microservices in a decoupled manner.
By leveraging these functionalities, developers can focus on building the core business logic within each microservice, streamlining the web development process and promoting code reusability.
Laravel Microservices Architecture
Imagine a large, monolithic application built with Laravel. While it might function well initially, as features and complexity grow, maintaining and scaling it becomes a challenge. This is where Laravel microservices architecture comes in.
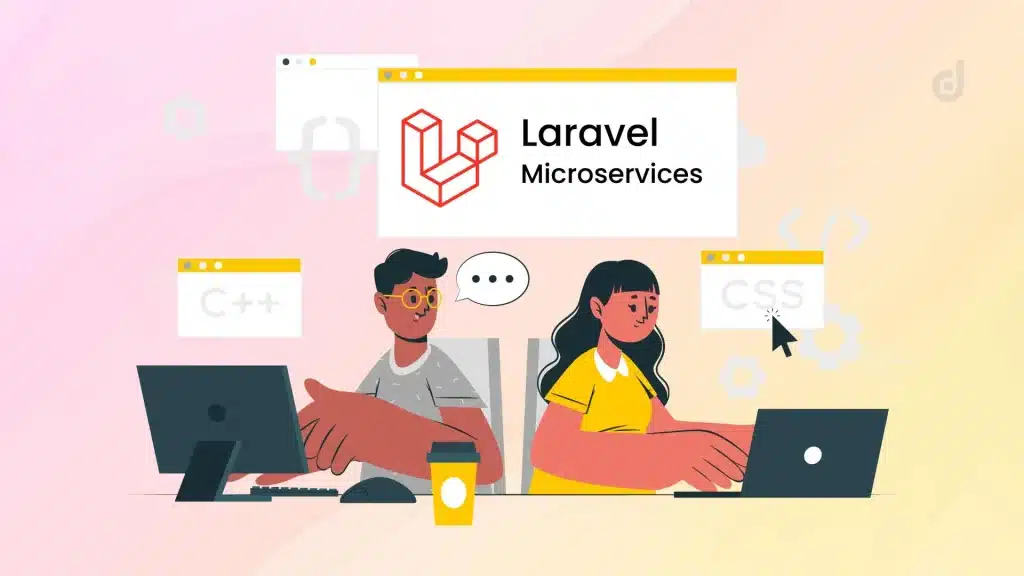
Laravel microservices architecture breaks down a complex application into smaller, independent services. Each service has a clearly defined responsibility, such as user management, product catalog, or order processing. These services communicate with each other through well-defined APIs, typically using protocols like REST.
Here are some key characteristics of Laravel microservices architecture:
1. Single Responsibility Principle
Each microservice within the architecture adheres to the Single Responsibility Principle. This means each service focuses on a single, well-defined functionality, like user authentication, product management, or order processing. This promotes code clarity and simplifies maintenance.
2. Loose Coupling
Tight dependencies between different parts of an application can lead to development bottlenecks. In Laravel microservices architecture, services communicate through well-defined APIs (Application Programming Interfaces). This loose coupling minimizes dependencies and allows for independent development and deployment of each service.
3. Independent Scalability
Not all parts of an application experience the same level of user traffic or growth. The beauty of microservices is that individual services can be scaled independently based on their specific needs. For instance, a high-traffic service handling product searches might require scaling up server resources, while a less frequently used service for managing user profiles could remain on a smaller server configuration.
Advantages of Laravel Microservices
The decision to adopt a microservices architecture for your Laravel application development can bring significant advantages. When combined with the strengths of the Laravel framework, these benefits become even more compelling. Here’s a look at some key advantages of leveraging Laravel microservices:
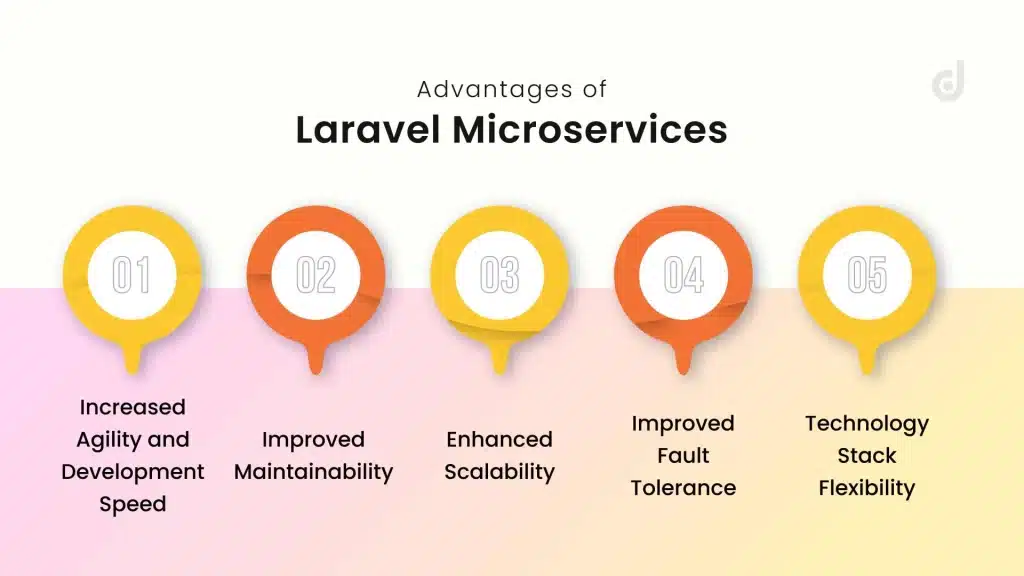
1. Increased Agility and Development Speed
Microservices break down complex applications into smaller, independent units. This allows development teams to work on individual services concurrently, leading to faster development cycles and quicker time-to-market for new features.
2. Improved Maintainability
Smaller, self-contained services are easier to understand, debug, and update compared to monolithic codebases. This translates to lower maintenance costs and a more manageable codebase in the long run.
3. Enhanced Scalability
As your application grows in user base and complexity, so too will its resource demands. The beauty of Laravel microservices is that you can scale individual services based on their specific needs. High-traffic services can be scaled up with additional resources, while less frequently used services can remain on a smaller configuration. This ensures optimal resource utilization and cost-effectiveness
4. Improved Fault Tolerance
The beauty of loosely coupled microservices is that a failure within one service has minimal impact on others. This fosters a more resilient application architecture, as errors are contained and don’t bring down the entire system.
5. Technology Stack Flexibility
The Laravel ecosystem offers a variety of tools beyond the core Laravel framework. Microservices architecture allows you to choose the most suitable technology for each service. For instance, you can leverage Laravel for complex services requiring a full-fledged framework, while opting for the lightweight Laravel Lumen framework for simpler services.
Overall, Laravel microservices empower developers to build applications that are not only feature-rich but also agile, scalable, and maintainable in the long run.
When Should You Opt for Laravel Microservices?
The decision to adopt a Laravel microservices architecture isn’t a one-size-fits-all approach. Here are some scenarios where Laravel microservices can be a powerful choice for your project:
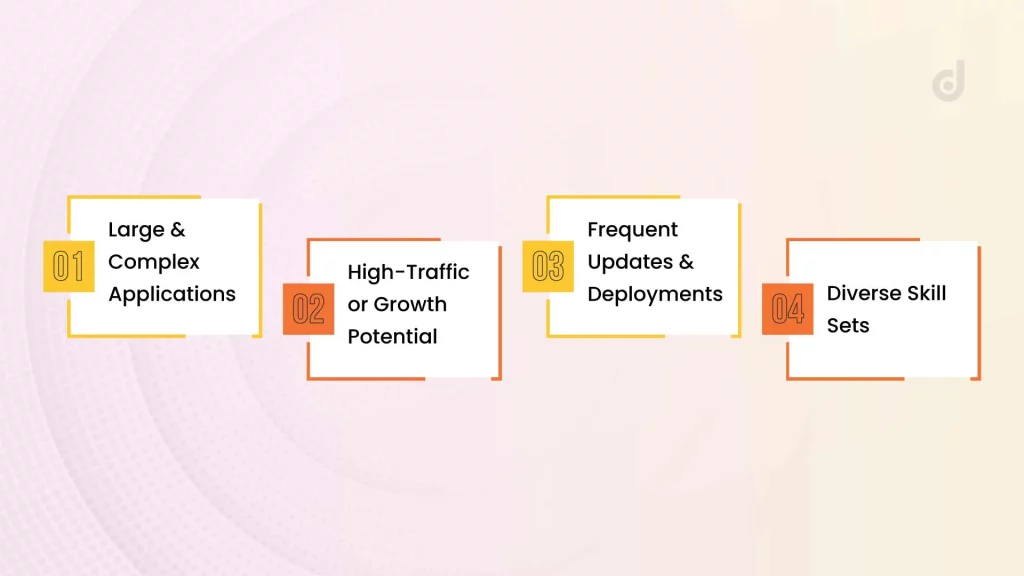
1. Large and Complex Applications
As applications grow in size and complexity, managing a monolithic codebase becomes increasingly challenging. Laravel microservices architecture shines in these situations, allowing you to decompose the application into smaller, more manageable units.
2. High-Traffic or Growth Potential
If your application experiences high user traffic or anticipates significant growth, microservices offer a scalable solution. Individual services can be scaled independently to handle surges in demand, ensuring a smooth user experience.
3. Frequent Updates and Deployments
Microservices architecture facilitates faster development cycles and easier deployments. If your application requires frequent updates and new features, this approach allows development teams to work on and deploy individual services independently.
4. Diverse Skill Sets
Microservices can empower teams with diverse skill sets. Developers can work on services written in different programming languages within the Laravel ecosystem (think Laravel vs. Lumen) based on their expertise.
However, it’s important to remember that microservices architecture also introduces additional complexity in terms of communication and coordination between services. For smaller, simpler applications, a well-structured monolithic approach might be sufficient.
Laravel Lumen
Laravel provides a powerful companion for building microservices: Laravel Lumen. Lumen is a lightweight framework specifically designed for developing APIs and microservices. Here’s why Lumen shines in this role:
- Focus on Core Functionality: Lumen removes many of the features found in the full Laravel framework, keeping its core focused on routing, middleware, and basic functionalities. This streamlined approach makes Lumen ideal for building lightweight microservices with minimal overhead.
- Faster Performance: Due to its focus on essentials, Lumen boasts faster performance compared to the full Laravel framework. This translates to quicker response times for your microservices.
- Easy Integration with Laravel Ecosystem: Lumen seamlessly integrates with other tools within the Laravel ecosystem, allowing you to leverage familiar libraries and packages within your microservices.
How to Build Microservices with Laravel Lumen?
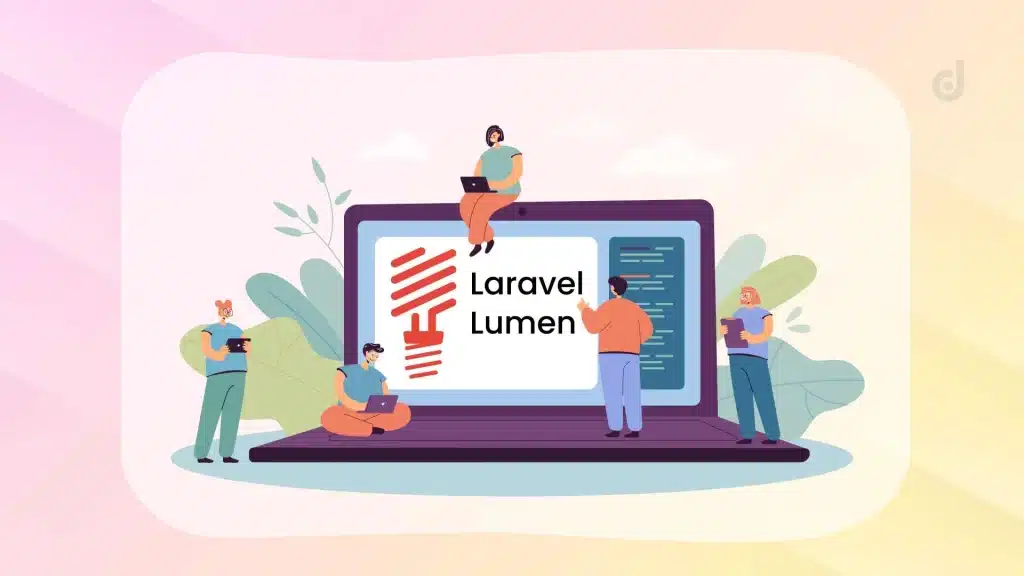
We’ve explored the theoretical aspects of Laravel microservices and the benefits of using Laravel Lumen for building them. Now, let’s get practical! Here’s a step-by-step guide to set up and build your first microservice with Lumen:
Step 1: Setting up a Lumen project
1. Prerequisites
Ensure you have Composer installed on your system. Composer is a dependency management tool essential for working with Laravel and Lumen projects.
2. Create a new Lumen project
Open your terminal and navigate to your desired project directory. Then, run the following command to create a new Lumen project named my-microservice:
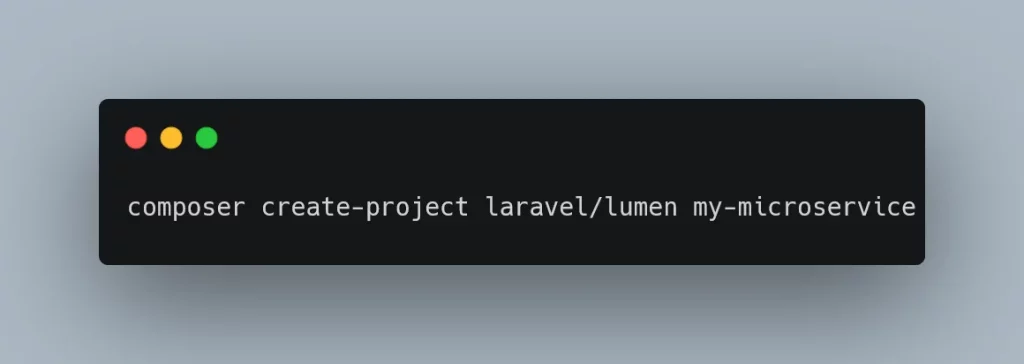
This command will use Composer to download and install the Lumen framework and its dependencies into the my-microservice directory.
3. Navigate to the project directory: Use the cd command to change your directory to the newly created project:
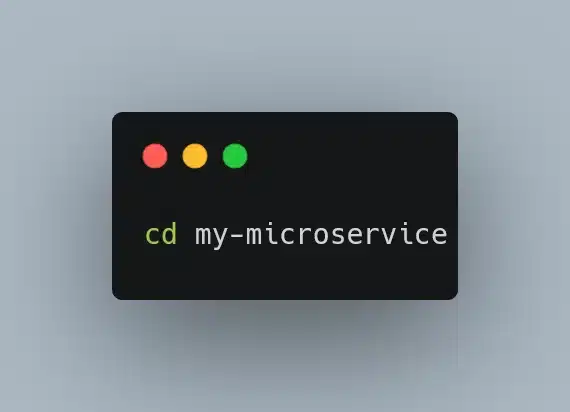
Now you have a basic Lumen project set up and ready for development.
Step 2: Defining Microservice Functionalities and APIs
Before diving into code, it’s crucial to define the purpose and functionalities of your microservice. This includes determining the specific tasks it will handle and the API endpoints it will expose for communication with other parts of your application.
Example: User Authentication Microservice
Let’s consider a simple example of a user authentication microservice built with Lumen. This service would be responsible for tasks like user registration, login, and password resets. Here’s a breakdown of its functionalities and potential API endpoints:
- Functionalities:
- User registration
- User login (including generating authentication tokens)
- Password reset functionality
- API Endpoints:
- POST /api/register – Endpoint for user registration with username, email, and password.
- POST /api/login – Endpoint for user login with username or email and password.
- POST /api/password/reset – Endpoint to initiate password reset process with user’s email address.
Documenting your API:
It’s essential to document your microservice’s API clearly. This documentation should specify details like:
- Endpoint URLs: The specific URLs for each API endpoint.
- HTTP Methods: The HTTP methods used for each endpoint (e.g., POST, GET, PUT).
- Request Parameters: The data expected in the request body or query parameters.
- Response Format: The structure of the data returned by the service in the response.
Having a well-defined API specification ensures smooth communication between your microservice and other components of your application.
Step 3: Implementing Business Logic Within Each Service
Now that you’ve defined the functionalities and API endpoints for your microservice, it’s time to translate them into code using Lumen. Here’s a breakdown of the steps involved:
1. Route Definition
Lumen’s routing system allows you to define how incoming API requests are mapped to specific controller methods within your service.
Here’s an example of defining the POST /api/register endpoint in Lumen’s routes/web.php file:
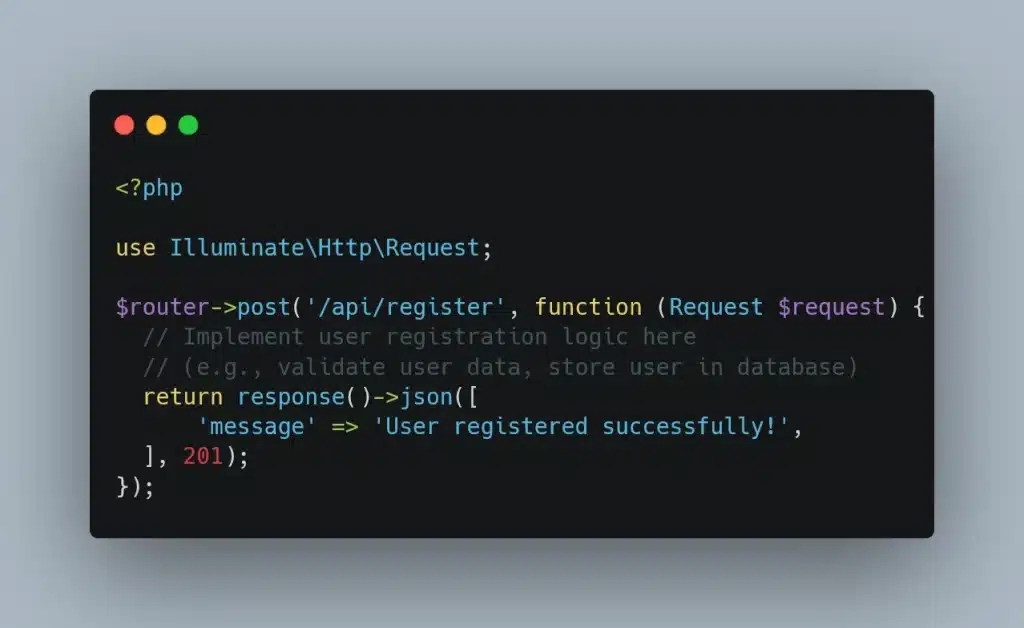
In this example, the POST /api/register route is mapped to an anonymous function that receives the incoming request object. Within this function, you’ll implement the business logic for user registration, such as validating user data and storing it in the database. The response object is then used to return a JSON response with a success message and a status code of 201 (Created).
2. Business Logic Implementation
The core logic for each functionality resides within the controller methods or dedicated service classes within your Lumen project. Here, you’ll leverage Lumen’s features or external libraries to handle tasks like:
- User data validation: Lumen provides built-in validation features or you can utilize libraries like Laravel’s Validator class.
- Database interaction: Lumen offers database access functionalities through its Eloquent ORM (Object-Relational Mapper).
- Password hashing: For secure password storage, consider using Laravel’s built-in password hashing functionalities.
By separating business logic from routing concerns, you maintain a clean and organized codebase within your microservice.
3. Error Handling
It’s crucial to handle potential errors gracefully within your microservice. Lumen provides exception handling mechanisms to return appropriate error responses with informative messages and HTTP status codes.
Note: This is a simplified example. The specific implementation details will vary based on your chosen functionalities and the complexity of your microservice’s business logic.
Step 4: Utilizing Laravel Features Like Queues and Events for Inter-Service Communication
While microservices advocate for loose coupling, communication between services is often necessary. Here’s how Lumen leverages Laravel’s built-in features to facilitate communication:
1. Queues
Asynchronous tasks are a common requirement in microservices. Lumen integrates seamlessly with Laravel’s queue system. This allows you to queue tasks like sending welcome emails after user registration or triggering notifications within separate worker processes. Here’s an example of dispatching a job to send a welcome email using Lumen’s queue functionality:
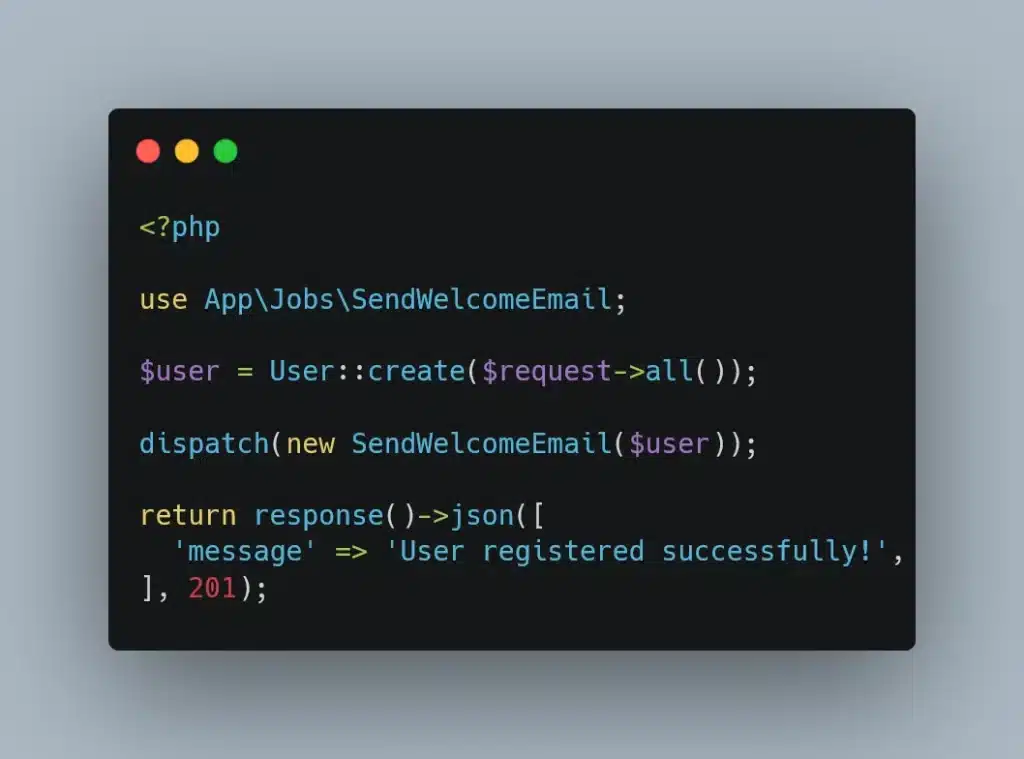
In this example, after registering a user, a SendWelcomeEmail job is dispatched to the queue. A separate worker process will then handle sending the email asynchronously, ensuring a smooth user registration experience.
2. Events
Laravel’s event system provides another powerful tool for inter-service communication. Events can be triggered within one microservice to notify other services about specific events, like a successful user registration or a password reset request. Here’s a simplified example:
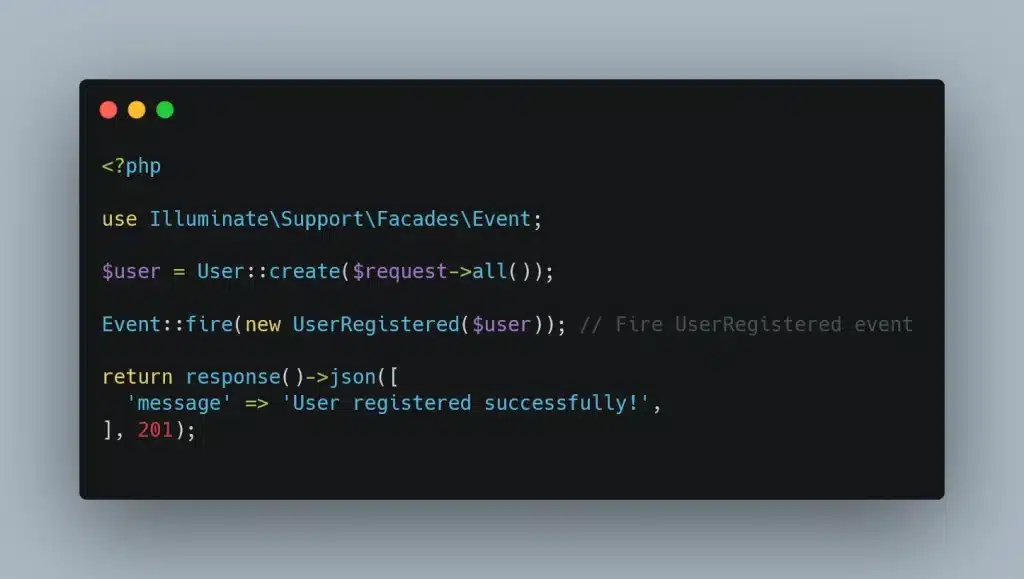
In this example, the UserRegistered event is fired after successful user registration. Other microservices can listen for this event and take appropriate actions, such as updating user profiles in a separate service.
By leveraging queues and events, Lumen empowers developers to build loosely coupled microservices that can communicate effectively without tight dependencies.
Step 5: Ensuring Reliability – Testing Your Laravel Microservices
Building robust microservices requires a comprehensive testing strategy. Here’s an overview of key testing approaches for Laravel microservices:
- Unit Tests: Unit tests focus on the smallest testable unit of code, typically an individual class or method within your microservice. Laravel’s built-in testing tools (PHPUnit) allow you to write unit tests that validate the functionality of individual components in isolation.
- Feature Tests: These tests focus on higher-level functionalities of your microservice, ensuring they behave as expected with various inputs and scenarios. Laravel’s Dusk browser testing framework can be used for feature tests that simulate user interaction with your microservice’s API endpoints.
- API Tests: Dedicated tools like Laravel Dingo API can be used to write comprehensive API tests that verify the behaviour and responses of your microservice’s endpoints under different conditions.
- Contract Testing: Contract testing focuses on the communication contract between microservices. Tools like Pact can be used to define and enforce the expected behaviour of your microservice’s API, ensuring compatibility with other services that depend on it.
By implementing a multi-layered testing approach, you can identify and address potential issues early in the development process, leading to more reliable and robust Laravel microservices.
Step 6: Deploying and Managing Individual Microservices
Once your Laravel microservices are built and tested, it’s time to deploy them to a production environment. Here are some key considerations:
- Independent Deployments: A significant advantage of microservices is the ability to deploy individual services independently. This allows for faster rollouts of new features or bug fixes in specific services without impacting the entire application.
- Deployment Options: There are various deployment options for your Laravel microservices. Popular choices include:
- Containerization: Containerization tools like Docker can package your microservices with all their dependencies into lightweight containers, ensuring consistent execution environments across different deployment platforms.
- Cloud Platforms: Cloud providers like AWS, Azure, and Google Cloud Platform offer managed services for deploying and scaling containerized applications.
- Monitoring and Logging: It’s crucial to monitor the health and performance of your microservices in production. Centralized logging solutions can help you track application behaviour and troubleshoot any issues that may arise.
Note: Managing a microservices architecture introduces additional complexity compared to monolithic applications. Investing in proper monitoring, logging, and deployment tools is essential for maintaining a healthy and scalable microservices ecosystem.
Conclusion
The world of web development is constantly evolving, and Laravel microservices architecture offers a compelling approach to building modern, scalable applications. By leveraging the strengths of Laravel and Laravel Lumen, you can decompose complex functionalities into smaller, independent services that are easier to develop, maintain, and scale.
This blog post has provided a roadmap for getting started with Laravel microservices. We’ve explored the core concepts, highlighted the advantages, and guided you through the steps of building a microservice with Laravel Lumen.
Ready to leverage the power of Laravel microservices for your next project?
Digimonk Solutions is a team of passionate Laravel developers with extensive experience in building scalable microservices architectures. We can help you design, develop, and deploy robust Laravel applications that meet your specific business needs.
Contact us today for a free consultation! Together, we can unlock the full potential of Laravel microservices and build applications that thrive in the ever-changing digital landscape.